初めに
前回 の続きです。
今回はNotion APIを利用する準備に取り掛かります。
APIで実行確認した後、Nextjsを利用するのでNotion SDKで利用できるようになるまでをゴールとします。
API準備
integration を作成
Notion APIを利用するためには、integrationというものを設定する必要があります。
利用する用途ごとにintegrationを作成し、Notionのdatabaseにintegrationからアクセスすることを許可することでAPIを利用することができるようになります。
integrationの作成は下記ページから。
流れに沿っていけば、すぐ作成できると思います。
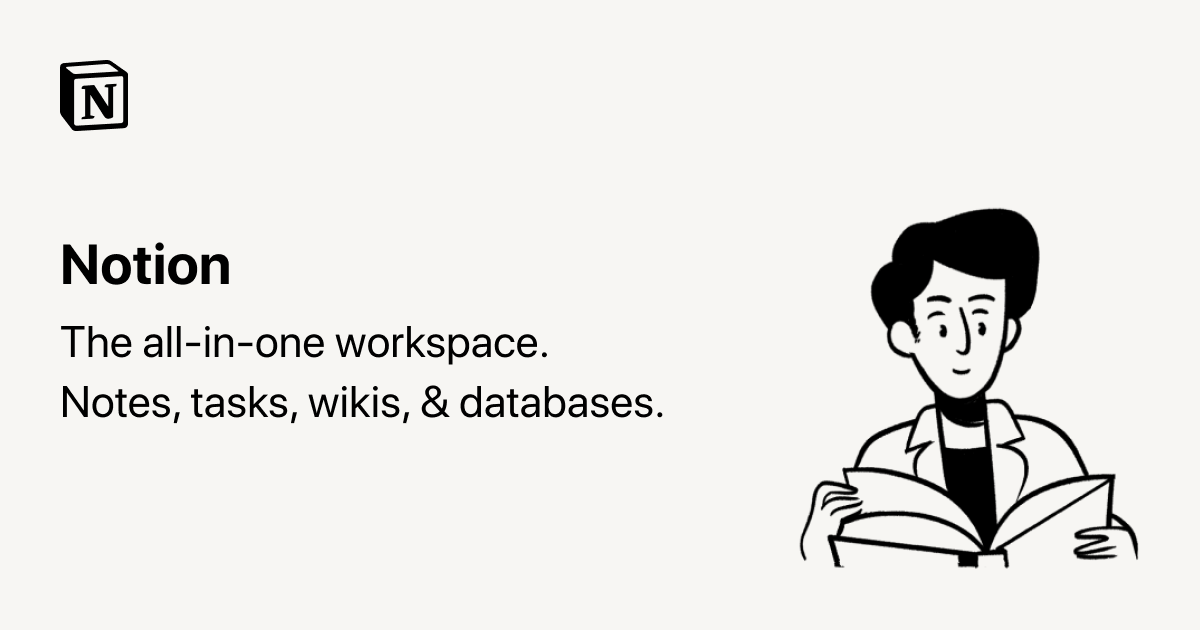
A new tool that blends your everyday work apps into one. It's the all-in-one workspace for you and your team
https://www.notion.so/my-integrations
💡
privateかpublicかどちらで利用するか設定する箇所があるので、間違えないようにしましょう
APIを使って記事の一覧を取得するためには、databaseに記事を入れておく必要があります。
よくNotion API でブログ化されている方は、databaseにtableを使用されることが多いのですが、
個人的に凄い管理しにくい...ズラッと並んでくれてたほうがわかりやすくね?
ってことで、list
を採用しています。
どちらでも問題ないと思いますが、APIで返ってくる型が変わるかどうかは未検証です。
databaseを作成したら画面右上のShare
から、作成したintegration
を指定して共有しましょう。
API実行
準備ができたので、とりあえずAPIを実行してみます。
Headは下記共通のよう。
1
2
Authorization: Bearer secret__xxxxx
Notion-Version: 2021-05-13
なお、レスポンス結果はめちゃくちゃ長いので割愛。
database内一覧取得
1
2
3
4
5
6
7
8
9
POST https://api.notion.com/v1/databases/{database_id}/query
{
"sorts": [
{
"timestamp": "last_edited_time",
"direction":"ascending"
}
]
}
ページ情報取得
1
GET https://api.notion.com/v1/pages/{block_id}
ページもブロックの一つと捉えるらしいので、block_id
にはpageのidを入れます。
子ブロック一覧取得
1
GET https://api.notion.com/v1/blocks/{block_id}/children
ページもブロックの一つと捉えるらしいので、block_id
にはpageのidを入れます。
Notion SDK
...と、ここまでAPIを実際に叩いて確認してきましたが、javascript / typescript に関しては、Notionがsdkを用意してくれています。
Official Notion JavaScript Client. Contribute to makenotion/notion-sdk-js development by creating an account on GitHub.
https://github.com/makenotion/notion-sdk-js
今回はNextjsで利用するので、ありがたくこのsdkを利用することにします。
1
yarn add @notionhq/client
使用してみる
Notion用のヘルパクラスを作ります。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
import { Client } from "@notionhq/client"
import type {
QueryDatabaseResponse,
ListBlockChildrenResponse,
} from '@notionhq/client/build/src/api-endpoints.d'
export default class Notion {
private _notion: Client;
private _token: string;
private _databaseId: string;
constructor(token: string, databaseId: string) {
this._token = token;
this._databaseId = databaseId;
this._notion = new Client({
auth: this._token
});
}
public async getPostList() {
const response: QueryDatabaseResponse = await this._notion.databases.query({
database_id: this._databaseId,
filter: {
and: [{
property: 'Published',
checkbox: { equals: true }
}],
},
sorts: [
{
"timestamp": "created_time",
"direction":"descending"
}
]
});
return postList;
}
public async getPostBlockListById(id: string) {
const response: ListBlockChildrenResponse = await this._notion.blocks.children.list({
block_id: id
});
return response;
}
}
とりあえず記事一覧と記事内子ブロック一覧の取得メソッドを作成。
一旦ガワだけ。
詳細は記事ページ作り込むときにでも。
仮の画面作成
とりあえず一覧画面と記事画面
作り込みは後日